[Шпаргалка] История версий PHP
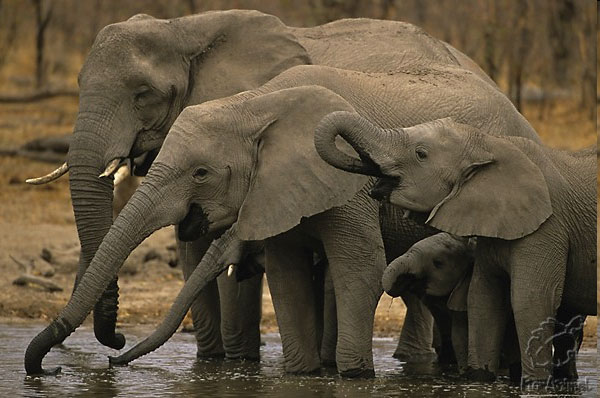
Периодически приходится работать с различными версиями PHP. И я постоянно забываю, что и когда можно использовать. Конечно, современные IDE подсказывают что нельзя использовать, но они не подсказывают что можно. Поэтому приходится постоянно лезть сначала в один changelog, потом в другой. В этой шпаргалке собраны воедино все фичи актуальных версий. Когда я говорю актуальных, то ссылаюсь на статистику Packagist.
5.3
- Closures
$lambda = function () {
echo 'Hello World!';
};
$lambda();
class myLambda
{
public function __invoke() {echo 'Hello World!';}
}
$lambda = new myLambda;
$lambda();
- Namespaces
- Short ternary
$first ?: $second;
- Labels:
start:
// do_something();
goto start;
- Garbage Collector
__callStatic
- Public Magic functions __get(), __set(), __isset(), __unset(), __call()
5.4
- Traits
- Short array syntax
$arr = [1, 2, 3];
- Array returned by function dereferencing
foo()[0];
switch ($arr) {
case [1, 2]:
}
- Closures $this
function () {
$this->doSomething();
}
- Class access on creation
(new Bar())->foo();
- Static call syntax with expressions
Class::{$foo . '_' . $bar}()
- Binary integers
0b0001
- Build-in WEB server
5.5
- Generators
finally
- list in foreach
$arr = [[1, 2]];
foreach ($arr as list($one, $two)) {
}
- Array and string dereferencing
[1, 2, 3][2];
"string"[1];
- Class fullname with
::class
namespace Foo\Bar;
class Baz {}
echo Baz::class; // Foo\Bar\Baz
5.6
- Constant expressions
class Some {
const A = 1;
const B = self::A + 1;
}
- Constant array
class A {
const AR = [1, 2, 3];
}
- Variadic function with argument unpacking
function name(...$args) {}
$args = [5, 'Yellow', true];
name(...$args);
- Exponential
**
use function
anduse const
__debugInfo
magic function
7.0
- Scalar types argument
- Return types
:type
- Null coalescing
isset($foo) ? $foo : $bar;
$foo ?? $bar;
- Spaceship operator
echo 1 <=> 1; // 0
echo 1 <=> 2; // -1
echo 2 <=> 1; // 1
- Constant array using define
- Anonymous classes
- Unicode escape symbols
echo "\u{9999}";
Closure::call($newthis)
- Grouped
use
use Foo\{Bar, Baz as Bar2};
- Generator return
yield from
intdiv
function- Throwable interface
- Variables of variables
PHP 5 PHP 7
$$foo['bar']['baz'] ${$foo['bar']['baz']} ($$foo)['bar']['baz']
$foo->$bar['baz'] $foo->{$bar['baz']} ($foo->$bar)['baz']
$foo->$bar['baz']() $foo->{$bar['baz']}() ($foo->$bar)['baz']()
Foo::$bar['baz']() Foo::{$bar['baz']}() (Foo::$bar)['baz']()
- foreach does not change internal array point
$array = [0, 1, 2];
foreach ($array as &$val) {
var_dump(current($array));
}
// 5: int(1) int(2) bool(false)
// 7: int(0) int(0) int(0)
7.1
- Nullable types
?int
void
return typeiterable
pseudo-type- Constant visibility modifiers
- List short syntax
- List key bindings
- Catching multiple exceptions types
Closure::fromCallable
7.2
- Abstract functions overloading
abstract class A { abstract function bar(stdClass $x); }
abstract class B extends A { abstract function bar($x): stdClass; }
- Argument type extending
- Trailing commas in namespaces
use A\B\{C,D,E,};
object
type hint
7.3
- Flexible Heredoc
foo(<<<HEREDOC
HEREDOC, true);
- Trailing commas in function call
JSON_THROW_ON_ERROR
is_countable
- Multibyte string improvements
7.4
- Typed properties
public int $count;
- Arrow functions
fn() => $this->x * 2
- Null coalescing assignment
// Before $data['date'] = $data['date'] ?? new DateTime();
$data['date'] ??= new DateTime();
- Scodead operator for array
$arr = [1, 2];
[0, ...$arr, 3, 4];
- FFI
- Preloading
- Covariant returns and contravariant parameters
interface A {
function m(B $z);
}
interface B extends A {
function m($z): A;
}
__serialize
,__unserialize
mb_str_split
- WeakRef
- Numeric literal separator
1_000_000_000;
- Deprecate left-associative ternary operator